Debugging SQLite DB using Drizzle Studio for Expo SQLite
When working with SQLite in an Expo project, debugging the database can be challenging. Thankfully, Drizzle Studio makes it easier by providing a user-friendly interface for inspecting and modifying your SQLite database. In this guide, we'll walk through setting up Drizzle Studio with Expo SQLite and demonstrate how to debug your database efficiently.
What is Drizzle Studio?
Drizzle Studio is a lightweight database inspector. It allows developers to view, query, and manage their database in a visual interface.
Setting Up Expo SQLite with Drizzle ORM
- Before integrating Drizzle Studio, ensure your project is set up with Expo and SQLite.
npx expo install expo-sqlite
- Install
expo-drizzle-studio-plugin
npm i expo-drizzle-studio-plugin
- Setup the plugin as shown:
import { useDrizzleStudio } from "expo-drizzle-studio-plugin";
import * as SQLite from "expo-sqlite";
import { View } from "react-native";
const db = SQLite.openDatabaseSync("db");
export default function App() {
useDrizzleStudio(db);
return <View></View>;
}
- Open devtools menu from the terminal with "start" process and choose
expo-drizzle-studio-plugin
shift + m
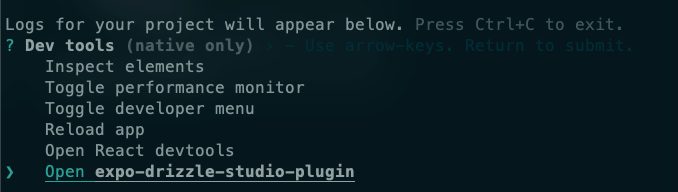
That's it. You can now view your emulator's SQLite db in your browser.
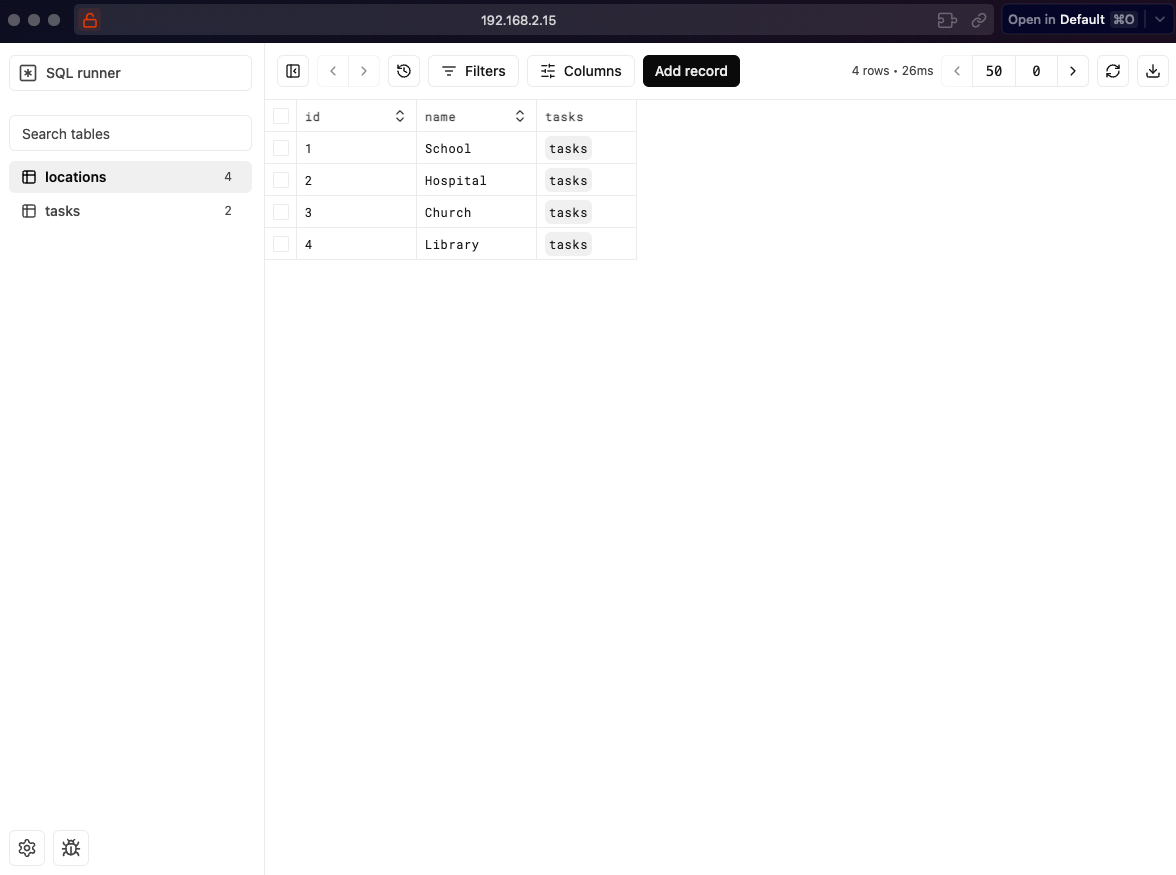
You even have the option to add records here and it will reflect on the app running on your device/emulator:
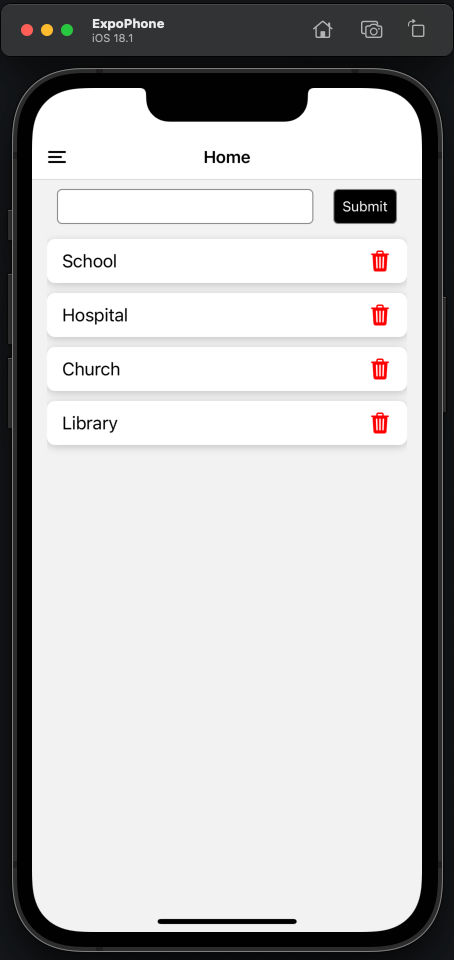
Pretty cool!